While Loops In Python- Python Tutorials For Beginners #11
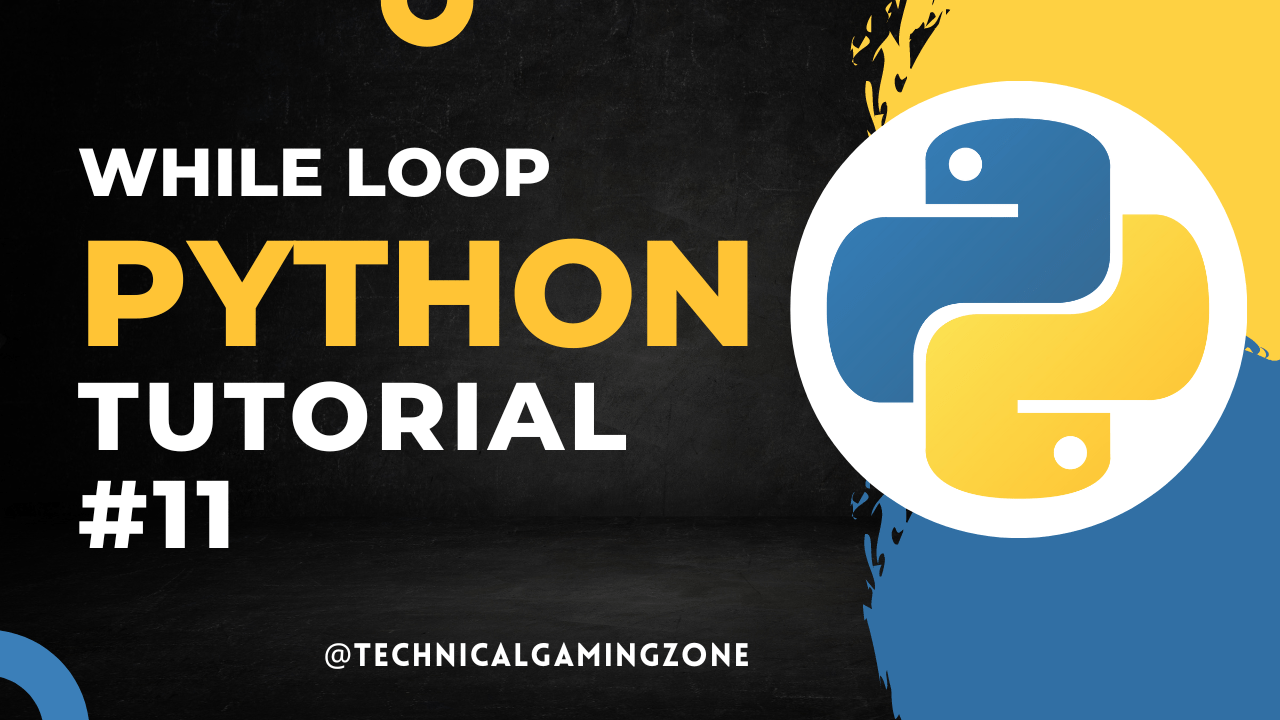
Overview
What Is The While Loops In Python ?
In Python, The WHILE LOOP works on the CONDITION given by the coder and the loop runs until the condition is TRUE. Whenever the condition becomes false, Then the loop will stop. So this is the mechanism of While Loop.
How To Create While Loops In Python ?
So first of all, We use the "while" Keyword to define the type of loop. After that, enter the condition in the parenthesis bracket or use space after the keyword. Both methods work exactly the same.
After the condition, Use a semicolon and press enter to enter in the code block of the while loop. Here, You will write a code that runs when the condition of the while loop is True, But it is important to check the Indentation of the code block. These are some practical examples that teach you way better than me.
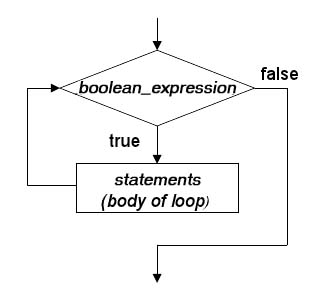
Condition:
Condition is a boolean expression. The loop continues to run as long as this condition evaluates to True.
Code Block:
Code Block is the set of statements that will be executed repeatedly if the contion is true. Make sure to update the variables involved in the condition within this block to eventually make the condition False, otherwise, you’ll create an infinite loop.
Examples Of While Loop
Example 1 : Infinite Loop
In this example, I created an infinite loop when I ran it, This code prints the "hello" word again and again until you close or kill your Python terminal. To create an infinite loop, I made an "a" variable whose value is 2 and I created a while loop. Here, (a==2) this condition is always True, There are no increments and decrements in the value of "a" so the value of Variable "a" will never change and conditions remain always true.
Example 2 : Finite Loop
This is a finite loop. After running the while loop the value of Variable "a" increases by 1. So, After some time the value of 'a' becomes more than 10 and the loop will stop, So this is a concept of a finite loop.
Example 3 : While Loop With Pass Keyword
1# Pass KeyWord
2
3while(a>=10):
4 pass
5
6# Pass Keyword works as placeholder in Loops & Functions
This is an example of a while loop with a pass keyword. The pass keyword works as a placeholder in functions and loops. With the help of pass keyword Python does not show any error because of incomplete loop. Now, we add code here whenever we want. Similarly, the pass keyword works in functions.
Example 4 : While Loop With Else Statements
1# While Loops With Else
2a = 0
3while(a<=2):
4 print('Hello')
5 a=a+1
6
7else:
8 print("Ended")
9
10# Else always runs for finite loops
We learnt about the if-else statement in previous blogs and the Else statement runs whenever the condition of while loops becomes false. Every time else statement will executes if the loop is finite.
What is the difference between a for and while loop?
For Loop
-
Initialization: Typically, the initialization is done within the loop statement.
-
Condition: The condition is checked before each iteration.
-
Update: The update statement is optional and executed after each iteration.
-
Use Case: Best used when the number of iterations is known in advance, such as iterating over a range or a collection.
Click Here To Learn More About For Loops In Python
While Loop
-
Initialization: The initialization is done outside the loop.
-
Condition: The condition is checked before each iteration.
-
Update: The update statement is typically inside the loop body and must be handled explicitly.
-
Use Case: Ideal when the number of iterations is not known beforehand and depends on a condition.
Key Differences
-
Scope of Initialization and Update: In a for loop, the initialization and update are part of the loop structure, while in a while loop, they are handled separately.
-
Flexibility: While loops offer more flexibility as they can handle more complex conditions and update statements.
Recommended Content:
- For Loops In Python- Python Tutorials For Beginners #9
- Project 2: Calculator - Python Tutorials For Beginners #8
- Match Cases - Python Tutorials For Beginners #7