Match Cases - Python Tutorials For Beginners #7
Overview
Introduction
Let’s dive into the world of Python’s match case statement. Introduced in Python 3.10, this feature provides a more concise and readable way to handle multiple conditions compared to traditional if-elif-else chains. Here’s what you need to know:
In this blog post, We will learn how to use match cases in Python, and see some examples of how they can simplify and improve your code.
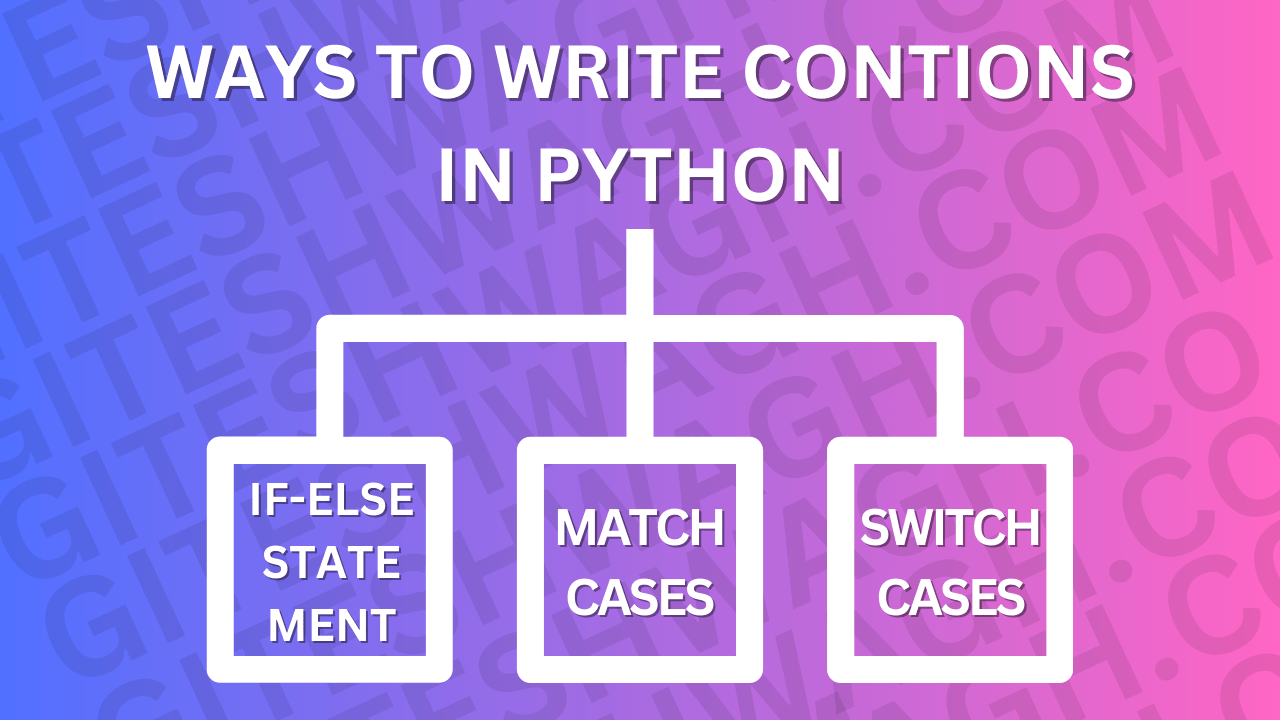
What Are Match Cases In Python?
Match Cases is a method or way for writing conditions in Python. Here, We create conditions related to the value of a variable. In If-Else statements, We define the condition with the help of conditional operators. But, In Match Cases the value of the variable is a condition. In Match Cases we program that, What will happen when the value of a variable is 1, 2, 3, etc... This is only for example. You will create a condition on any value of variable.
Is Match Case Same As Switch Case?
In Python, Match Case And switch Cases Are Not Same, They are Similar in that they both allow you to Compare A Value against different cases and execute different blocks of code depending on the match.
However, Match Case Is More Powerful And Expressive Than Switch Case, because it can do the following things that switch case cannot:
-
Match on types, attributes, and patterns, not just literals and constants.
-
Use ranges, bindings, guards, and wildcards to make the cases more flexible and concise.
-
Be used as an expression that returns a value, not just a statement that performs an action.
-
Ensure that the cases are exhaustive and cover all possible values, or raise an exception otherwise.
In other languages, Match cases are similar to Switch Cases, But they are More Powerful And Expressive. They allow you to match a value or an expression against different patterns, and execute different blocks of code depending on the match.
Syntax Of Match Cases
1match expression:
2 case pattern1:
3 # code block for pattern1
4 case pattern2:
5 # code block for pattern2
6 # ...
7 case patternN:
8 # code block for patternN
9 case _:
10 # default code block
Examples of Match Cases
This a simple example of match cases.
1x = input("Enter the number : ")
2# variable x with the input system
3
4match x:
5 # Condition 1
6 case '1':
7 print("The value is 1")
8
9 # Condition 2
10 case '2':
11 print("The value is 2")
12
13 # Condition 3
14 case '3':
15 print("The value is 3")
16
17 # Condition 4
18 case '4':
19 print("The value is 4")
20
21 # Else Condition
22 case _:
23 print("other value")